Testing gRPC Endpoints: How to Test API Endpoints for Vulnerabilities
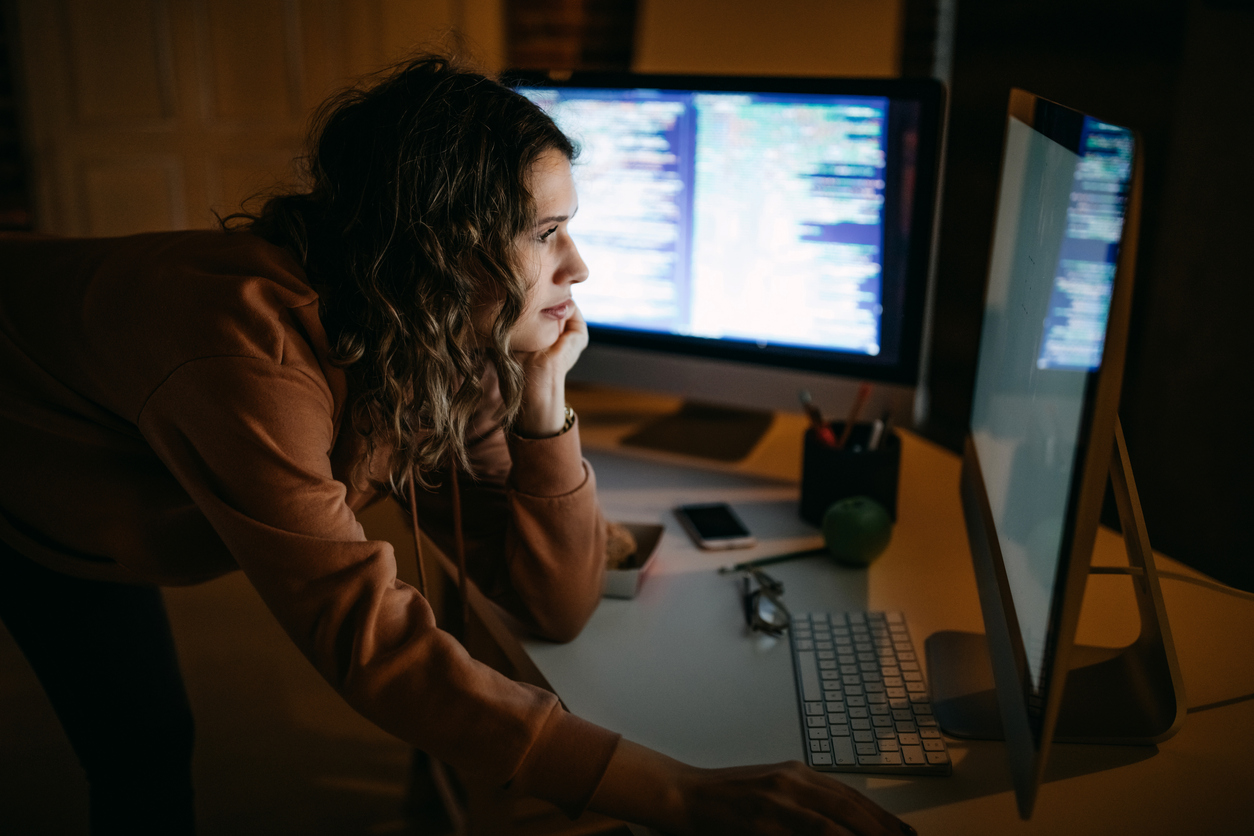
As an API developer, you need to ensure your API endpoints are secure and protected from vulnerabilities. Failing to properly test API security can have serious consequences – like data breaches, unauthorized access, and service disruptions.
This blog post will provide you with practical guidance on best practices for testing the security of API endpoints, including a step-by-step technical example of how to test gRPC endpoints. It outlines the types of security testing that should be performed and the different types of security vulnerabilities that can be found in API endpoints and provides tips for remediation. By following the guidance in this article, you can build a robust security testing plan for your API endpoints.
OWASP API Security Checklist: The Types of Tests to Perform
To ensure the security of your API endpoints, you should perform several types of testing, as recommended in the OWASP API Security Checklist. By performing these types of tests regularly, you can gain assurance that your API endpoints are secure and address any vulnerabilities that are identified to protect your APIs and your consumers.
- Penetration testing examines API endpoints for vulnerabilities that could allow unauthorized access or control. This includes testing for injection flaws, broken authentication, sensitive data exposure, XML external entities (XXE), broken access control, security misconfigurations, and insufficient logging and monitoring.
- Fuzz testing, or fuzzing, submits invalid, unexpected, or random data to API endpoints to uncover potential crashes, hangs, or other issues. This can detect memory corruption, denial-of-service, and other security risks.
- Static application security testing (SAST) analyzes API endpoint source code for vulnerabilities. This is useful for finding injection flaws, broken authentication, sensitive data exposure, XXE, and other issues early in the development lifecycle.
- Dynamic application security testing (DAST) tests API endpoints by sending HTTP requests and analyzing the responses. This can uncover issues like injection, broken authentication, access control problems, and security misconfigurations.
- Abuse case testing considers how API endpoints could potentially be misused and abused. The goal is to identify ways that the API could be used for malicious purposes so that appropriate controls and protections can be put in place.
{{api-cta}}
Common API Vulnerabilities and How to Test for Them
To ensure the security of your API endpoints, you must test for common vulnerabilities. Some of the major issues to check for include:
- SQL injection: This occurs when malicious SQL statements are inserted into API calls. Test for this by entering ' or 1=1;-- into API parameters to see if the database returns an error or additional data.
- Cross-site scripting (XSS): This allows attackers to execute malicious JavaScript in a victim's browser. Try entering into API parameters to check for reflected XSS.
- Broken authentication: This allows unauthorized access to API data and functionality. Test by attempting to access API endpoints with invalid or missing authentication credentials to verify that users are properly authenticated.
- Sensitive data exposure: This occurs when API responses contain personally identifiable information (PII) or other sensitive data. Review API responses to ensure no sensitive data is returned.
- Broken access control: This allows unauthorized access to API resources. Test by attempting to access API endpoints with different user roles or permissions to verify proper access control is in place.
Testing gRPC Endpoints: A Technical Example
Integration Testing gRPC Endpoints in Python
To ensure your gRPC API endpoints are secure, you should perform integration testing. This involves sending requests to your API and analyzing the responses to identify any vulnerabilities.
Step 1
First, use a tool like Postman, Insomnia, or BloomRPC to send requests to your gRPC server. Test all endpoints and methods in your API.
- Set up a gRPC channel and stub to connect to the server.
- Call the appropriate gRPC methods on the stub to send requests and receive responses.
Step 2
Next, analyze the responses for information disclosure. Make sure that no sensitive data is returned in error messages or stack traces.
- In the test_endpoint_1 function, send a request to Endpoint 1.
- Handle any exceptions that occur during the request and analyze the error message or status code for potential information disclosure.
Step 3
Then, test for broken authentication by sending requests without authentication credentials. The API should return a “401 Unauthorized” status code.
- In the test_endpoint_2 function, send a request to Endpoint 2 without providing authentication credentials.
Catch any grpc.RpcError exceptions that occur and check the error code to ensure that it is “401 Unauthorized.”
Step 4
Finally, execute the tests. Call the test methods you have defined in your script to execute the integration tests:
It is important to note that gRPC API endpoint testing has many variations depending on the programming language and technologies you are using. For the example given above, there are many extension possibilities.
For instance, you can further expand the code and add more test methods for other steps such as testing access control, handling malformed requests, checking TLS encryption, and reviewing API documentation for any discrepancies with the actual implementation.
Ongoing API Endpoint Security Testing Best Practices
To ensure that API endpoints remain secure over time, ongoing security testing is essential. Schedule regular vulnerability scans and penetration tests to identify any weaknesses that could be exploited.
Conduct Regular Vulnerability Scans
Run automated vulnerability scans on API endpoints at least monthly. Scan for issues like:
- SQL injection
- Cross-site scripting
- Broken authentication
- Sensitive data exposure
Remediate any critical or high-severity findings immediately. Develop a plan to address medium- and low-severity issues within 30-90 days.
Perform Penetration Tests
Have an independent third party conduct penetration tests on API endpoints every 6-12 months. Penetration tests go deeper than vulnerability scans to simulate real-world attacks. Testers will attempt to access sensitive data or take control of the API. Address any issues found to strengthen endpoint security.
Monitor for Anomalous Behavior
Continuously monitor API endpoints for abnormal behavior that could indicate compromise or abuse. Look for things like:
- Sudden spikes in traffic
- Requests from unknown or suspicious IP addresses
- Invalid requests or requests attempting to access unauthorized resources
Investigate anything unusual immediately to determine if remediation is needed. Monitoring is key to quickly detecting and responding to security events.
Review Access Controls
Review API endpoint access controls regularly to ensure that only authorized users and applications can access data and resources. Remove any unused, outdated, or unnecessary permissions to limit exposure. Access controls are a critical line of defense, so keeping them up-to-date is important for security.
Conclusion
In conclusion, testing the security of API endpoints should be an ongoing process to protect systems and data. By following best practices for identifying vulnerabilities through various types of security testing, you can remediate issues and strengthen endpoint security over time.
While the examples above focused on gRPC endpoints, the overall guidelines apply to any API. Regularly testing API endpoints is key to avoiding breaches and ensuring the integrity of your infrastructure. Make security testing a priority and keep your endpoints protected.